Setup ad insertion
This guide explains how to enable ad insertion workflow and ad tracking with SmartLib, including interaction with third party ad libraries.
During these steps, you will learn:
- how to initialize parameters to perform personalization of the ad insertion.
- how to configure SmartLib to collect ads consumption on the end user’s device.
If the ad provider is Google, you will have to follow additional steps to integrate the Google PAL SDK.
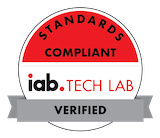
SmartLib ad tracking is IAB certified and OM SDK compliant
Follow OM SDK integration to enable the viewability and verification measurement.
- Follow Project setup
- Follow Session handling
- Follow Player integration
- A player that supports ad tracking
1. Activate advertising
At this point, you should have initialized the lib, created a streaming session object and have integrated the session lifecycle into your app.
In order to activate the ad insertion workflow (ad session creation, ad tracking and ad analytics), it is required to call this method after creating the streaming session object.
- Android
- iOS & tvOS
- Web
/**
* Activate advertising workflow (including analytics and tracking)
* The method setAdParameter automatically calls this method
*/
session.activateAdvertising();
/**
* Activate advertising workflow (including analytics and tracking)
* The method setAdParameter automatically calls this method
*/
[session activateAdvertising];
/**
* Activate advertising workflow (including analytics and tracking)
* The method setAdParameter automatically calls this method
*/
session.activateAdvertising();
The session has to be started with the getURL
method and must not be done on the main thread ! Because of generation duration of the Google nonce and technical requirements, it has to be done on another thread.
2. Set session ad parameters
Ad parameters are sent to the BkYou and the ad provider to generate a more personalized ad insertion. They have to be set before each streaming session with ads and are reset once used.
- Android
- iOS & tvOS
- Web
/**
* This method set a parameter used for the ad insertion
* @param name Parameter name
* @param value Parameter value
*/
session.setAdParameter("name", "value");
/**
* This method set a parameter used for the ad insertion
* @param name Parameter name
* @param value Parameter value
*/
[session setAdParameter:@"name" value:@"value"];
/**
* This method set a parameter used for the ad insertion
* @param name Parameter name
* @param value Parameter value
*/
session.setAdParameter("name", "value");
3. Listen to ad events
This method allows the app to listen to ad events:
- when an ad break begins,
onAdBreakBegin
is triggered - when an ad break ends,
onAdBreakEnd
is triggered - when an ad begins,
onAdBegin
is triggered - when an ad ends,
onAdEnd
is triggered - if an ad is skippable,
onAdSkippable
is triggered afteronAdBegin
with skip begin position, current ad end position and ad break end position. This allows to skip either the current ad or all ads in ad break.
It can be used to interact with the UI, for instance to lock player controls during an ad or show a skip button.
The placement of the ad break can be computed:
- pre-roll: the ad break position is equal to 0
- post-roll: the ad break end position (ad break position + ad break duration) is greater than the content duration - 1 second
- mid-roll: all other cases
Find more details about AdData
and AdBreakData
in Ad options.
Since the version 04.06.02, modifications have been made to the arguments of the ad events listener. Please update your code if needed.
Here are the modifications that have been implemented:
position
➡adBreakData.startPosition
oradData.startPosition
duration
➡adBreakData.duration
oradData.duration
clickURL
➡adData.clickURL
sequenceNumber
➡adData.index
totalNumber
➡adBreakData.adCount
- Android
- iOS & tvOS
- Web
session.setAdEventsListener(new AdManager.AdEventsListener() {
/**
* Triggered when ad breaks begin
* @param adBreak Ad break data
*/
void onAdBreakBegin(AdBreakData adBreak) {
// Lock player controls
}
/**
* Triggered when an ad begin
* @param adData Ad data
* @param adBreakData Ad break data
*/
void onAdBegin(AdData adData, AdBreakData adBreakData) {
// Show ad link button if needed
}
/**
* Triggered when an ad is skippable
* @param adData Ad data
* @param adBreakData Ad break data
* @param adSkippablePosition position in millis where skip becomes allowed
* @param adEndPosition position in millis of ad end
* @param adBreakEndPosition position in millis of ad break end
*/
void onAdSkippable(AdData adData, AdBreakData adBreakData, long adSkippablePosition, long adEndPosition, long adBreakEndPosition) {
// Show the skip message/button "skip ad in x seconds"
}
/**
* Triggered when the ad is ended, not called if skipped
* @param adData Ad data
* @param adBreakData Ad break data
*/
void onAdEnd(AdData adData, AdBreakData adBreakData) {
// Hide ad link, hide ad skip button
}
/**
* Triggered when ad breaks ended, even in case of skipping
* @param adBreakData Ad break data
*/
void onAdBreakEnd(AdBreakData adBreakData) {
// Unlock player controls, hide ad link, hide ad skip button
}
});
// Registering to ad events
[session setAdEventsListener:self];
/**
* Triggered when ad breaks begin
* @param adBreak Ad break data
*/
- (void)onAdBreakBegin:(AdBreakData *)adBreak {
// Lock player controls
}
/**
* Triggered when an ad begin
* @param adData Ad data
* @param adBreakData Ad break data
*/
- (void)onAdBegin:(AdData *)adData adBreakData:(AdBreakData *)adBreakData {
// Show ad link button if needed
}
/**
* Triggered when an ad is skippable
* @param adData Ad data
* @param adBreakData Ad break data
* @param adSkippablePosition position in millis where skip becomes allowed
* @param adEndPosition position in millis of ad end
* @param adBreakEndPosition position in millis of ad break end
*/
- (void)onAdSkippable:(AdData *)adData adBreakData:(AdBreakData *)adBreakData adSkippablePosition:(long)adSkippablePosition adEndPosition:(long)adEndPosition adBreakEndPosition:(long)adBreakEndPosition {
// Show the skip message/button "skip ad in x seconds"
}
/**
* Triggered when the ad is ended, not called if skipped
* @param adData Ad data
* @param adBreakData Ad break data
*/
- (void)onAdEnd:(AdData *)adData adBreakData:(AdBreakData *)adBreakData {
// Hide ad link, hide ad skip button
}
/**
* Triggered when ad breaks ended, even in case of skipping
* @param adBreakData Ad break data
*/
- (void)onAdBreakEnd:(AdBreakData *)adBreakData {
// Unlock player controls, hide ad link, hide ad skip button
}
session.setAdEventsListener({
/**
* Triggered when an ad break begin
* @param adBreakData Ad break data
*/
onAdBreakBegin: (adBreakData) => {
// Lock player controls
},
/**
* Triggered when an ad begin
* @param adData Ad data
* @param adBreakData Ad break data
*/
onAdBegin: (adData, adBreakData) => {
// Show ad link button if needed
},
/**
* Triggered when an ad is skippable
* @param adData Ad data
* @param adBreakData Ad break data
* @param adSkippablePosition position in millis where skip becomes allowed
* @param adEndPosition position in millis of ad end
* @param adBreakEndPosition position in millis of ad break end
*/
onAdSkippable: (adData, adBreakData, adSkippablePosition, adEndPosition, adBreakEndPosition) => {
// Show the skip message/button "skip ad in x seconds"
},
/**
* Triggered when the ad is ended, not called if skipped
* @param adData Ad data
* @param adBreakData Ad break data
*/
onAdEnd: (adData, adBreakData) => {
// Hide ad link, hide ad skip button
},
/**
* Triggered when ad break ended, even in case of skipping
* @param adBreakData Ad break data
*/
onAdBreakEnd: (adBreakData) => {
// Unlock player controls, hide ad link, hide ad skip button
}
});
The method has to be called before starting the session (i.e calling getURL
).
4. Send click tracking event
This method notifies the lib that the user clicked on the ad. If the ad provider defined a tracking callback, it will be called. The application should manage the URL independently, such as opening the web browser, while the lib is responsible for the tracking.
It has to be called during the playback of the ad.
- Android
- iOS & tvOS
- Web
// Send ad click when the user opens the ad link
session.adUserInteraction(AdInteractionType.CLICK);
// Send ad click when the user opens the ad link
[session adUserInteraction:BPAdInteractionTypeClick];
// Send ad click when the user opens the ad link
session.adUserInteraction('click');
5. What's next?
-
If you are using Google as ad provider, follow Google integration.
-
If you need the ad data before starting the playback,
- To perform a UI specific behavior, follow Listen to ad data
- To implement a bookmark management, follow Create a bookmark position
6. Full example
- Android
- iOS & tvOS
- Web
// Init SmartLib and Google PAL
SmartLib.getInstance().init(getApplicationContext(), ..., ..., ...);
// Create a new session
StreamingSession session = SmartLib.getInstance().createStreamingSession();
// Attach the player
session.attachPlayer(player);
// Listen to ad events
session.setAdEventsListener(new AdManager.AdEventsListener() {
@Override
public void onAdBreakBegin(AdBreakData adBreak) {
// Lock player controls
mControlsLayout.setClickable(false);
}
@Override
public void onAdBegin(AdData adData, AdBreakData adBreakData) {
// Show ad link button if needed
mAdLink.setVisible(View.VISIBLE);
mAdLink.setClickURL(adData.clickURL); // on click, it will call session.adUserInteraction(AdInteractionType.CLICK);
}
@Override
public void onAdSkippable(AdData adData, AdBreakData adBreakData, long adSkippablePosition, long adEndPosition, long adBreakEndPosition) {
// Show the skip message/button "skip ad in x seconds"
mAdSkipMessage.setVisibility(View.VISIBLE);
}
@Override
public void onAdEnd(AdData adData, AdBreakData adBreakData) {
// Hide ad link and ad skip button
mAdLink.setVisible(View.GONE);
mAdSkipMessage.setVisibility(View.GONE);
}
@Override
public void onAdBreakEnd(AdBreakData adBreakData) {
// Hide ad link and ad skip button
mAdLink.setVisible(View.GONE);
mAdSkipMessage.setVisibility(View.GONE);
// Unlock player controls
mControlsLayout.setClickable(true);
}
});
...
// Start the session with/without ads
if (contentContainsAds) { // To be adapted
session.activateAdvertising();
// Set ad parameters
session.setAdParameter("name1", "value1");
session.setAdParameter("name2", "value2");
}
// You must not call getURL on the main thread !
// Because of generation duration of the nonce and technical requirements, it has to be done on another thread
StreamingSessionResult result = session.getURL(CONTENT_URL);
...
// Notify that the user opened the ad link
session.adUserInteraction(AdInteractionType.CLICK);
// Stop the session
session.stopStreamingSession();
...
// Init SmartLib
[SmartLib initSmartLib:... nanoCDNHost:... broadpeakDomainNames:...];
// Init PAL SDK (if using Google)
[[AdManager sharedManager] setPalParameters:descriptionURL
partnerName:partnerName
partnerVersion:partnerVersion
omidVersion:omidVersion
playerType:playerType
playerVersion:playerVersion
ppid:ppid
videoPlayerHeight:videoPlayerHeight
videoPlayerWidth:videoPlayerWidth
willAdAutoPlay:willAdAutoPlay
willAdPlayMuted:willAdPlayMuted];
// Create a session
StreamingSession *session = [SmartLib createStreamingSession];
// Attach the player
[session attachPlayer:player];
// Listen to ad events
[session setAdEventsListener:self];
...
// Start the session with/without ads
if (contentContainsAds) { // To be adapted
[session activateAdvertising];
[session setAdView:player.view]
// Set ad parameters
[session setAdParameter:@"name1" value:@"value1"];
[session setAdParameter:@"name2" value:@"value2"];
}
// Start the session
StreamingSessionResult *result = [session getURL:CONTENT_URL];
...
// Notify that the user opened the ad link
[session adUserInteraction:BPAdInteractionTypeClick];
// Stop the session
[session stopStreamingSession];
...
// Init SmartLib
SmartLib.getInstance().init(...);
// Attach PAL SDK (if using Google)
AdManager.getInstance().attachPALSDK(goog);
// Set consent settings (if using Google)
const isConsentToStorage = getConsentToStorage();
const consentSettings = new goog.pal.ConsentSettings();
consentSettings.allowStorage = isConsentToStorage;
AdManager.getInstance().setConsentSettings(consentSettings);
// Set Google PAL parameters at init (if using Google, to speed up first nonce generation)
AdManager.getInstance().setPalParameters(descriptionURL, partnerName, partnerVersion,
omidVersion, playerType, playerVersion, ppid,
videoPlayerHeight, videoPlayerWidth, willAdAutoPlay, willAdPlayMuted);
// Create a session
const session = SmartLib.getInstance().createStreamingSession();
// Attach the player
session.attachPlayer(player);
// Listen to ad events
session.setAdEventsListener({
onAdBreakBegin: (adBreakData) => {
// Lock player controls
},
onAdBegin: (adData, adBreakData) => {
// Show ad link button if needed
},
onAdSkippable: (adData, adBreakData, adSkippablePosition, adEndPosition, adBreakEndPosition) => {
// Show the skip message/button "skip ad in x seconds"
},
onAdEnd: (adData, adBreakData) => {
// Hide ad link, hide ad skip button
},
onAdBreakEnd: (adBreakData) => {
// Unlock player controls, hide ad link, hide ad skip button
}
});
...
// Start the session with/without ads
if (contentContainsAds) { // To be adapted
session.activateAdvertising();
// Set ad parameters
session.setAdParameter('name1', 'value1');
session.setAdParameter('name2', 'value2');
}
// Start the session
session.getURL(CONTENT_URL)
.then(result => {
...
});
...
// Notify that the user opened the ad link
session.adUserInteraction('click');
...
// Stop the session
session.stopStreamingSession();
...